Deitel C++ How To Program 9th Edition Pdf Free Download
- Deitel C++ How To Program 9th Edition Pdf Free Download Windows 7
- Deitel C++ How To Program 9th Edition Pdf Free Download Free
For Introduction to Programming (CS1) andother more intermediate courses covering programming in C++. Alsoappropriate as a supplement for upper-level courses where theinstructor uses a book as a reference for the C++ language.
Deitel C++ How To Program 9th Edition Pdf Free Download Windows 7
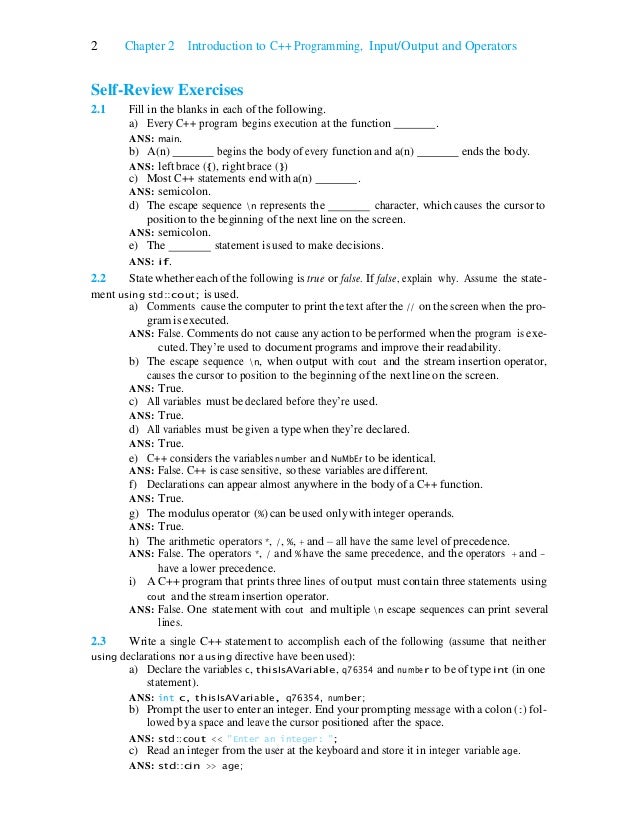
- C++ How to Program (9th Edition) [Paperback] [Paul Deitel] on Amazon.com. Get your Kindle here, or download a FREE Kindle Reading App.
- Ebook C How To Program (8th Edition) By Paul Deitel;Harvey Deitel.PDF. Deitel, Harvey M. Deitel, 8th Edition. 28th International Symposium. Related eBooks Java How To Program 8th International Edition By Harvey M Deitel Paul J Deitel.
ارائه پهنای باند اختصاصی به شرکت ها و سازمان ها ارائه خدمات پهنای باند و راهکارهای ارتباطی پرشین گیگ به شما کمک میکند تا از امکانات بهتری نسبت به روشهای اشتراکی مانند xDSL جهت ارسال و دریافت داده ها بهره مند شوید.
This best-selling comprehensive text isaimed at readers with little or no programming experience. Itteaches programming by presenting the concepts in the context offull working programs and takes an early-objects approach. Theauthors emphasize achieving program clarity through structured andobject-oriented programming, software reuse and component-orientedsoftware construction. The Ninth Edition encourages students toconnect computers to the community, using the Internet to solveproblems and make a difference in our world. All content has beencarefully fine-tuned in response to a team of distinguishedacademic and industry reviewers. Hindi hd movies 1080p free download.
Deitel C++ How To Program 9th Edition Pdf Free Download Free
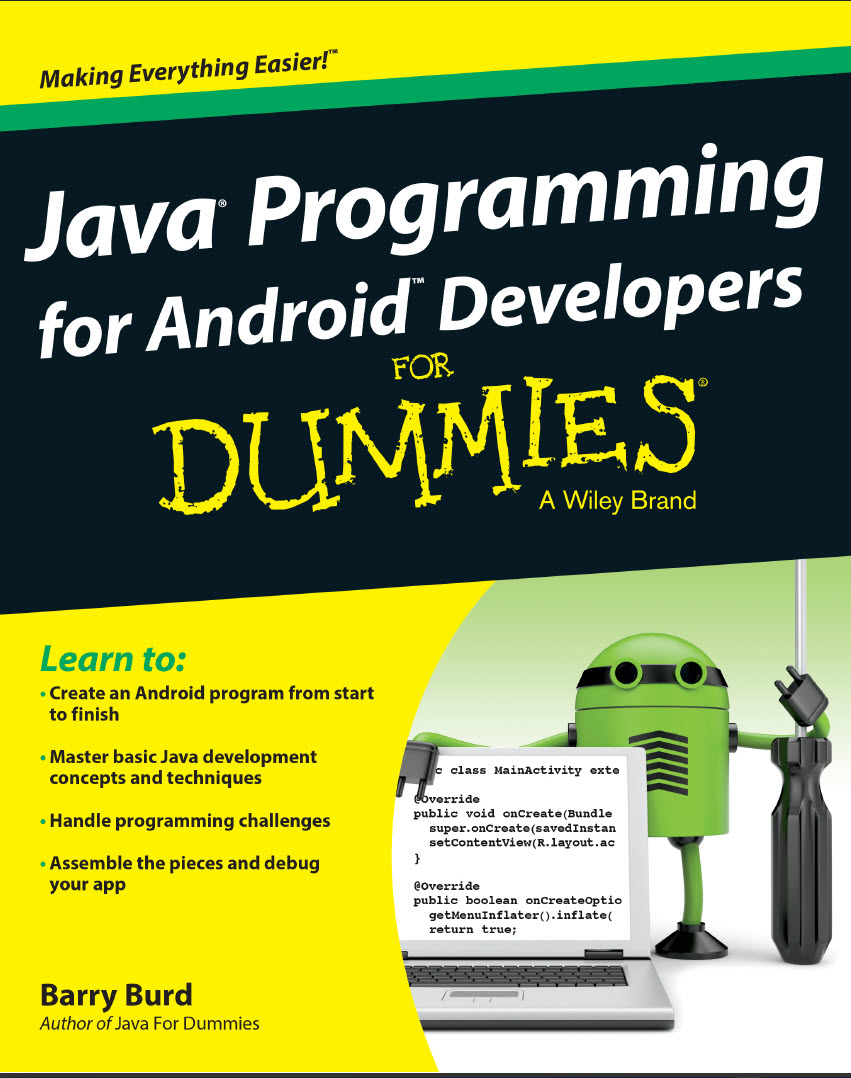
MyProgrammingLabforC++ How to Program¿ is a total learning package.MyProgrammingLab isan online homework, tutorial, and assessment program that trulyengages students in learning. It helps students better prepare forclass, quizzes, and exams–resulting in better performance inthe course–and provides educators a dynamic set of tools forgauging individual and class progress. And, MyProgrammingLab comesfrom Pearson, your partner in providing the best digital learningexperience.
Apr 9, 2018 - Ingenico iCT250 USB drivers. Ingenico iCT220 firmware; Ingenico iCT250 accessories; Ingenico iCT250 Driver; Ingenico iwl250 USB drivers. The iCT250 is the innovative payment solution that's so smart, it stands alone. It accepts all existing forms of electronic payment – including NFC/contactless,. Ingenico ict220 driver usb.
Note: If you are purchasing thestandalone text or electronic version, MyProgrammingLab doesnot come automatically packaged with the text. To purchaseMyProgrammingLab, please visit: myprogramminglab.com or youcan purchase a package of the physical text + MyProgrammingLab bysearching the Pearson Higher Education web site.¿MyProgrammingLab is not a self-paced technology and should only bepurchased when required by an instructor.
Subprocess post installation script cydia 7. View the Deitel Buzz online to learn more aboutthe newest publications from the Deitels.